Some old computer languages are destined to never die. They do, however, evolve. For example, Fortran, among the oldest of computer languages, still has adherents, not to mention a ton of legacy code to maintain. But it doesn’t force you to pretend you are using punched cards anymore. In the 1970s, if you wanted to crunch numbers, Fortran was a good choice. But there was another very peculiar language: APL. Turns out, APL is alive and well and has a thriving community that still uses it.
APL has a lot going for it if you are crunching serious numbers. The main data type is a multidimensional array. In fact, you could argue that a lot of “modern” ideas like a REPL, list types, and even functional programming entered the mainstream through APL. But it did have one strange thing that made it difficult to use and learn.
[Kenneth E. Iverson] was at Harvard in 1957 and started working out a mathematical notation for dealing with arrays. By 1960, he’d moved to IBM and a few years later wrote a book entitled “A Programming Language.” That’s where the name comes from — it is actually an acronym for the book’s title. Being a mathematician, [Iverson] used symbols instead of words. For example, to create an array with the numbers 1 to 5 in it and then print it, you’d write:
⎕←⍳5
Since modern APL has a REPL (read-eval-print loop), you could remove the box and the arrow today.
What Key Was That?
Wait. Where are all those keys on your keyboard? Ah, you’ve discovered the one strange thing. In 1963, CRTs were not very common. While punched cards were king, IBM also had a number of Selectric terminals. These were essentially computer-controlled typewriters that had type balls instead of bars that were easy to replace.
With the right type ball, you could have 26 upper-case letters, 10 digits, a few control characters, and then a large number of “weird” characters. But it is actually worse than that. The available symbols were still not numerous enough for APL’s appetite. So some symbols required you to type part of the symbol, press backspace, then type more of the symbols, sometimes repeating the process several times. On a printing terminal, that works fine. For the CRTs that would soon take over, this was tough to do.
For example, a comment (like a REM in Basic or a // in C++) is represented by a thumbnail (⍝). In other words, this would be an APL comment:
⍝ This is a comment
To make that character, you’d type the “arch” part, backspace, then the “dot” part. Not very speedy. Not very practical on old CRT terminals, either.
The characters aren’t the only strange thing. For example, APL evaluates math right to left.
That is, 3×2+5 is 21 because the 2+5 happens first. You just have to get used to that.
A Solution
Of course, modern screens can handle this easily and most people use an APL keyboard mapping that looks like your normal keyboard, but inserts special symbols when you use the right Alt key (with or without the shift modifier). This allows the keyboard to directly enter every possible symbol.
Of course, your keyboard’s keycaps probably don’t have those symbols etched in, so you’ll probably want a cheat sheet. You can buy APL keycaps or even entire keyboards if you really get into it.
What’s GNU With You?
While there have been many versions of APL over the years, GNU APL is certainly the easiest to setup, at least for Linux. According to the website, the project has more than 100,000 lines of C++ code! It also has many modern things like XML parsers.
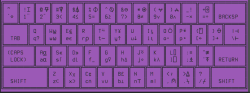
The real trick is making your keyboard work with the stranger characters. If you are just playing around, you can consider doing nothing. You can see the keyboard layout by issuing the ]KEYBD command at the APL prompt. That will give you something like the adjacent keyboard layout image.
From that image, you can copy and paste odd characters. That’s a pain, though. I had good luck with this command line:
setxkbmap -layout us,apl -variant ,dyalog -option grp:switch
With this setup, I can use the right alt key to get most APL characters. I never figured out how to get the shifted alternate characters, though. If you want to try harder, or if you use a different environment than I do, you might read the APL Wiki.
An Example
Rather than do a full tutorial, here’s my usual binary search high low game. The computer asks you to think of a number, and then it guesses it. Not the best use of APL’s advanced math capabilities, but it will give you an idea of what it can do.
Here’s a survival guide. The upside-down triangle is the start or end of a function. You already know the thumbnail is a comment. A left-pointing arrow is an assignment statement. A right-pointing arrow is a goto (this was created in the 1960s; modern APL has better control structures, but they can vary between implementations). Square boxes are for I/O, and the diamond separates multiple statements on a single line.
∇ BinarySearchGame ⍝ Initialize variables lower ← 1 upper ← 1024 turns ← 0 cheating ← 0 ⍝ Start the game 'Think of a number between 1 and 1024.' ⋄ ⎕ ← '' Loop: turns ← turns + 1 guess ← ⌊(lower + upper) ÷ 2 ⍝ Make a guess using binary search ⍞ ← 'Is your number ', ⍕ guess, '? (h for high, l for low, c for correct): ' response ← ⍞ → (response = 'c')/Finish ⍝ Jump to Finish if correct → (response = 'h')/TooHigh ⍝ Jump to TooHigh if too high → (response = 'l')/TooLow ⍝ Jump to TooLow if too low → InvalidInput ⍝ Invalid input TooHigh: upper ← guess - 1 → (lower > upper)/CheatingDetected ⍝ Detect cheating → Loop TooLow: lower ← guess + 1 → (lower > upper)/CheatingDetected ⍝ Detect cheating → Loop InvalidInput: ⍞ ← 'Invalid input. Please enter "h", "l", or "c".' ⋄ ⎕ ← '' turns ← turns - 1 ⍝ Invalid input doesn't count as a turn → Loop CheatingDetected: ⍞ ← 'Hmm... Something doesn''t add up. Did you make a mistake?' ⋄ ⎕ ← '' cheating ← 1 → Finish Finish: → (cheating = 0)/Continue ⍝ If no cheating, continue → EndGame Continue: ⍞ ← 'Great! The number is ', ⍕ guess, '. It took ', ⍕ turns, ' turns to guess it.' ⋄ ⎕ ← '' EndGame: ⍞ ← 'Would you like to play again? (y/n): ' restart ← ⍞ → (restart = 'y')/Restart ⍝ Restart the game if 'y' → Exit ⍝ Exit the game otherwise Restart: BinarySearchGame ⍝ Restart the game Exit: ⍞ ← 'Thank you for playing!' ⋄ ⎕ ← '' ⍝ Exit message ∇
What’s Next?
If you want to get an idea of how APL’s special handling of data make some programs easier, the APL Wiki has a good page for that. If you don’t want to install anything, you can run APL in your browser (although it is the Dyalog version, a very common choice for modern APL).
If you don’t want to read the documentation, check out [phoebe’s] video below. We always wanted the IBM computer that had the big switch to go from Basic to APL.
APL Keyboard image via Reddit
In high school we had an IBM system card eating system with the selectric terminal.
When we loaded APL, we would replace the type ball for the selectric with one with the APL character set, and had plastic keyboard overlay that had the keys marked. You would check the system light panel to see what shift level you were in.
We did the reverse in college. After RSTS/E got support for 2741-type terminals (134.5 baud), we borrowed the type ball from our Secretary’s Selectric typewriter after hours and used it instead of the APL type ball on an APL terminal, normally used on the computer center’s Burroughs system, so that we could generate nice output (vs. dot matrix) with upper- and lower-case.
Array based programming is great! I’ve become a big fan of Uiua (https://www.uiua.org/), a modern experimental language that takes a lot of influence from APL and its descendants.
The MCM/70 was an early Canadian made desktop computer with built in APL.
https://hackaday.io/project/184646-mcm70-reproduction
Ken Iverson taught APL to me and my classmates in 1967, in high school. The symbol you call “thumbnail” was called “lamp” by Mr. Iverson. It looks a bit like the schematic symbol for an (incandescent) light bulb, and the idea was that it was “illuminating” text. I loved APL, but never used it again, save for taking a class in it at Cornell U. in 1972. After that, it’s been “normal” languages that companies would pay me to write. Oh – almost forgot: there was an implementation of APL for CPM, believe it or not. Didn’t cover the entire language, but it worked. I modified a Synertek CRT terminal to display the APL character set by replacing the character generator ROMs.
Thanks for spelling out REPL!
I wondered what it meant when reading a recent post by [Arya].
There’s always Google, but I prefer the Wikipedia page on the idea. Lots of interpreted languages have REPLs. One of the nice things about interactive BASIC on old microcomputers was that it was essentially a REPL environment. https://en.wikipedia.org/wiki/Read%E2%80%93eval%E2%80%93print_loop
I think it’s what we used to call a “command line”!
I don’t. A commandline is basically used for launching programs, while REPL is for interacting with a language environment, setting variables, single-stepping and so on.
I think it would be fair to say that the commandline facility of Bash is a subset of its REPL.
J (https://www.jsoftware.com/#/README) is an APL variant that uses ASCII rather than special symbols. Iverson was one of its developers. It doesn’t have the “I wrote code in Martian” flair of APL, or the fun of keyboard-map hacking, but it might be more convenient if you just want to write APL-style code.
I’ve never used J myself, and only ever played around with APL.
25 years ago i had a summer job to maintain some old fortran code and none of the scientists wanted to explain to me the purpose, i was just supposed to make it work the same on this modern linux box as it did on the old dec something sitting beside it.
it was an exemplar of “a ton of legacy code” — quite massive, more than i could read in the month that i was on the project. but what i found in the end is that it was just a histogram, and the bulk of the code was layered hacks to deal with long-gone I/O hardware. the rest of it was a genuinely awful user interface — again, mostly centered on controlling the complex I/O rat’s nest. if they’d just told me they wanted a histogram, i feel like i could have come up with something much simpler / smaller / more maintainable / usable.
i wonder how much of “ton of legacy code” is made up of these projects with 10,000+ lines of code, all of it obsolete except for a trivial 10-line math kernel.
In 1994 Mathworks had a 30,000+ line set of installation scripts for MATLAB. I sent support an email, “I don’t know whether to laugh or cry. Some people write operating systems in fewer lines.”
I instrumented the scripts to store a time stamp when they ran. Many were called multiple times because no one knew how the mess worked. So they just added more bandaids.
I learned Fortran, Pascal, Cobol and assembler during my studies at uni but I remember I could never run a Fortran program properly. And I never used Fortran after that.
Fortunately none of our teachers had the crazy idea to inflict APL on us. Otherwise it would surely have started a riot.
Btw I wonder if there is a computer language based on hieroglyphics. If not, it must have been an oversight. ;)
I learned APL in high school (early ’70s). Being a math geek, I was fascinated. Was CS major in college (used it when I could). Hired by IBM into an APL shop maintaining production code in support of our salesmen (territory & quota tracking). Eventually moved on to PL/I, REXX, DB2, Pipelines (VM environment).
Still use APL today; tinkering/prototyping. Very fast matrix/array manipulation. Prefer the GitHub version; clickable characters on a control bar of your workspace screen.
APL is only funny-looking and exotic for people who never studied computer science or treated their technical university as vocational school that’s mostly supposed to teach them building corporate CRUD in C#, Java or Python.
Excellent retro intro to APL from 1975, complete with teleprinter https://youtube.com/watch?v=_DTpQ4Kk2wA
APL/360 was the first computer language I learned and I loved it. The character set is part of the charm. I even used APL/1130 with the “cryto-shift” keyboard. To inlcude all the the characters on the IBM 1130 console it used a keyboard with and upper-left and upper-right shift. BTW, what you call a “thumbnail” I know as a “lamp” which I find is a better name for a comment indicator.
APL – the world’s first “write-only” language.
FORTRAN was not “one of the oldest” (high level) programming languages, it is in fact the oldest such PL. Before FORTRAN came out in 1956 and revolutionized programming, people were essentially working directly in machine code.
I do not know how the REPL came into the mainstream, but the concept presumably originated in LISP (which, having been defined in 1958, usually is considered the second high level PL).
Well, Backus did “Speedcoding” before Fortran which is one of those things that is usually lumped as “Autocode” — you can debate if those were real languages and they certainly were machine specific that I know of. Whirlwind had the L&Z system, UNIVAC had ARITH_MATIC about the same time, too. IPL was out before LISP, but wasn’t very successful. There were a bunch that beat FORTRAN I out the door like COMTRAN, for example.
I had to look but FORTRAN I was in 1957 (the concept goes back to 1955). LISP was dreamed up around 1958 but the first implementation was in 1959 around the same time as JOVIAL (which survived thanks to the military), ALGOL, and a bunch of other forgotten ones.
So it is one of those things… it is first if you define the term one way. Not so much if you define it another way. That’s why I said “one of the” — it really wasn’t germane to my point to get into the semantics of it. Personally, I miss SNOBOL sometimes ;-)
LISP may have been defined in 1958, but as far as I can tell it wasn’t implemented until 1960. ALGOL was implemented in 1958.
I use Polish language and need Alt-GR
(like french, czech, spanish etc)
how survive?
I fondly recall a book by Gerrit A. Blaauw titled Digital System Implementation (1976) in which he used APL to describe computer hardware/architecture. Hardware description languages were a bit of a novelty at the time (at least to me).
50-some years ago I could touch-type APL. And now I wonder how long muscle memory lasts.
Fortran: “But it doesn’t force you to pretend you are using punched cards anymore” … Sometimes it does, if there is legacy code from that era mixed in. I used this recollection in 1998 to aid a fellow student in a molecular modeling class who couldn’t understand why her code wouldn’t run (the exercise was to add several lines to an existing program, which was in F77).
I learned Fortran on punch cards back in 1976, and remember how excited we were when they installed terminals that let you enter code on a terminal. Not exactly sure if it could actaully be called a terminal, because it was a keyboard that typed what you typed onto greenbar paper. It was interactive, because somehow it stored your code on the system, and you executed it with what sure looked like a terminal command. A year or two later, I was writing code on a black/green terminal connected to the mainframe with (gasp) a 300 baud modem. The future!
And now I carry a computer in my pocket faster than the first 6 or 8 computers I owned. The future!!!
I don’t speak APL myself but it has always fascinated me. Nobody has mentioned what seems to me the best APL trick of all: getting Conway’s game of life to run in just one line of code!
life ← {⊃1 ⍵ ∨.∧ 3 4 = +/ +⌿ ¯1 0 1 ∘.⊖ ¯1 0 1 ⌽¨ ⊂⍵}
See here:
https://aplwiki.com/wiki/Conway%27s_Game_of_Life
I can’t read this now, but I did pore over it and work it all out once.
Dyalog (who are one of the biggest commercial APL vendors) have an excellent presentation on how it works:
https://www.youtube.com/watch?v=a9xAKttWgP4
Every time I watch it I can almost delude myself that I understand how it works.
I learned APL in the late 1970s on a DECwriter (the one with shifted Greek characters in .. red .. on the keyboard) on a DECsystem 20.
It bent my brain permanently and beneficially. I had never seen such diverse dimensionality of operands and results, and the generalization of inner and outer products to any binary operations that you could imagine were literally mind-expanding. As I write here about it I also recall the arbitrarily powerful data projection operations where you could select or drop data for the next operation. I found it surfacing again unbidden in my head when I was doing data analysis in PostgreSQL.
The character set was extremely important, to me anyway. I recall having a hard time with the weird little escapes that they used on terminals that did not have a character set that could represent it: I think they were ADM3as and ADM31s. A single character rho is not in any way equivalent to seeing “.rho” embedded in your statement.
I used APL when I was a grad student in the early 1980’s. The terminals we had were Tektronix 4010 graphic terminals with storage tubes, so the backspace-and-overprint trick worked pretty much the same as ink on paper, except that you would press a key to erase everything at once after you filled the page.
I used it mainly for numerical solution of differential equations.
Those Tektronix 4006 and 4010 terminals were wonderful alien technology: high resolution green line vector graphics that could imitate a raster device. I spent a few days on an escape sequence based Tengwar font for it, just because glowing Elvish letters …
The DECwriter accomplished the overprint function with the backspace key, and after a short delay the printhead would pull far enough to the .. right, I think it was, so you could see what you had created. Hrrrgh, it said, each time: I remember the small sound.
I learned APL as a kid in the 70s. When I visited my big brother at work, he would sit me down at a terminal and start up the APL tutorial. I completed many levels despite never having heard of half the math it was doing; the tutorial made it all seem very straightforward. When I got to college I was disappointed to learn no other language had a built-in matrix inversion operator. It did make for an interesting résumé, though, when I applied to work at the college computer center: “Languages? BASIC and APL.”
For those who want to dabble in APL without investing too much cash or a custom keyboard, consider these APL key cap stickers: https://www.tindie.com/products/russtopia/apl-keyboard-symbol-sticker-set/
Disclaimer: I made ’em :)
They’re durable and work for laptop or desktop keyboards. Compatible with GNU APL, Dyalog (a commercial variant) and probably others like NARS2000.
I was /aware/ of APL at university in the late 70s, although possibly only because it was mentioned in one of the early DDJs. Much more recently I’ve used it to post-process database (PostgreSQL) output, plus for some ad-hoc maths.
I wrote my own 2741 (i.e. Selectric-based terminal) emulator to handle the IBM 1130 variant (and later others), and to be frank feel that video terminals were a significant factor in the practical demise of the language.
It’s /far/ easier to remember the position of a couple of dozen basic characters, many of which appear on the same key as a tenuously-related letter, and then to compose using the backspace key, than it is to remember a hundred or so with four characters per key.
Consider https://web.archive.org/web/20200403045314im_/http://www.aplusdev.org/keyboard.gif, and weep.
What was modern about the take? Complaining about unfamiliar characters is as old as writing itself. In particular, APL symbols have names, including lamp (for elucidation). Double printing lives on in text based formatting, such as how underlining and bold text work in man pages. Double typing also, in character combining, often simplified to dead keys. Alternate graphics sets are common for non-US locales, e.g. {[]} all require AltGr in the current standard Swedish keyboard layout (and vice versa, our letters do in the eu layout). Many other languages go for multigraphs instead, e.g. === in php or ..= in rust.
I bet the “>” isn’t part of the original APL code ;).
Damn, I meant >;
No!!! >&;
Damn, can’t get it right. Oh well, you know what I mean.
Interestingly, the symbol based syntax reminds me a lot of emojicode.
As a native speaker of APL (my first computer language, thanks to Explorer Post 76 in Owego NY), it’s always good to see people talking about it. I drop mention of it from time to time and like to pull it out for white-boarding one-line solutions when interviewing.
When I switched from engineering to computer science in the early 70’s, Fortran IV was the version in use. Last year, for grins, I searched for Fortran in use on GitHub; Fortran 90 is not recognizable to me.
As a student employee, I wrote an online editor in APL that did data validation for data entry to a database.
Perl has been described as a write-only language, meaning that once it’s written that it’s non decipherable language. I think that APL tops Perl in trying to figure out what it does.
I learned APL in 1976, starting with York APL and then IBM APL. Most of the time I worked on an Anderson Jacobsen modified Selectric trrminal, but also a Tektronix 4010 terminal with APL character ROM. The 4010 was a storage type CRT which wrote to a fine mesh and illuminated the screen with a flood gun projected through the mesh. What was cool is that the mesh could be read by raster scanning the mesh and looking at the modulated beam current. Thst signal could be amplified and sent to a static plotter. It was big stuff to be able to get an instant screen print from a graphics CRT terminal I. 1976.
Oh my god I have an IBM model with these weird symbols on it and I’ve been wondering for years what they might be they are APL symbols!
Did my linear algebra class problem sets in APL when in college. We had one Tektronix storage tube terminal, several 2741 Selectric terminals (almost always broken) and, later, some ASR-38 wide carriage, upper/lower case Teletypes with APL fonts. I, however, had a Model 33, and for us, there were escape characters, so “inverted triangle DEL” was $DEL (or something). This was APL on the Control Data CYBER 74, running KRONOS timesharing at UMASS/Amherst in the 70s.
There were actually TWO typeballs for the 2741, depending on whether your terminal was “EBCDIC” or “Correspondence” code. Using the wrong typeball made things even more interesting.