Blockchains claim to be public, distributed, effectively immutable ledgers. Unfortunately, they also tend to get a little bit huge – presently the Bitcoin blockchain is 194GB and Ethereum weighs in at 444GB. That poses quite an inconvenience for me, as I was looking at making some fun ‘Ethereum blockchain aware’ gadgets and that’s several orders of magnitude too much data to deal with on a microcontroller, not to mention the bandwidth cost if using 3G.
Having imagined a thin device that I could integrate into my mobile phone cover (or perhaps… a wallet?) dealing with the whole blockchain was clearly not a possibility. I could use a VPS or router to efficiently download the necessary data and respond to queries, but even that seemed like a lot of overhead, so I investigated available APIs.
As it turns out, several blockchain explorers offer APIs that do what I want. My efforts get an ESP8266 involved with the blockchain began with two of the available APIs: Ethplorer and Etherscan.
Where Blockchain and Microcontroller Collide
To start with something simple, I set out to build a small gadget that contains a hardcoded Ethereum address, retrieves the Eth balance at that address over Wi-Fi using the Etherscan API, and displays it on a small OLED screen (without requiring any of your security keys of course).
In a few days I’ll expand on this with an application of the websockets protocol to create a simple automated service controller. Imagine for example a conference room that you can rent with Ethereum, where power to the room is supplied for a period of time depending on how much Eth is sent to an address. In other words, an Ethereum-controlled switch with a timer!
As usual I’ll be using a NodeMCU board (I really did order a lot of them) which uses an ESP8266, but there’s no reason this would be difficult to achieve on an Arduino-based device. I chose a 128×32 pixel OLED screen based on the SSD1306 controller. I really like this line of screens because they are high contrast and easy to read, come in a number of form factors, are inexpensive, and are easy to use as long as you choose the right NodeMCU compile options.
Pros and Cons of the API Options
Ethplorer (Github) responds with data to HTTP GET requests. Some key features are that it allows you to retrieve trading data like pricing and volume for both coins and tokens. Price tracking gadgets have been done before, and this looks like a great way to implement it.
Etherscan on the other hand focuses on more things that I’m interested in. It has some basic smart contract features like checking execution status, and in addition to HTTP GET requests it supports websockets for something approaching real-time alerting.
Both services are presently free to use and can check Eth balances, transactions histories, and the other features you’d expect in this type of blockchain explorer. I chose Etherscan for this project because I wasn’t interested in price data and I thought of some fun things websockets would allow. Both services provide data in JSON format, which is quite convenient as we’ll see later.
Preparing the Firmware is Easy with NodeMCU-build
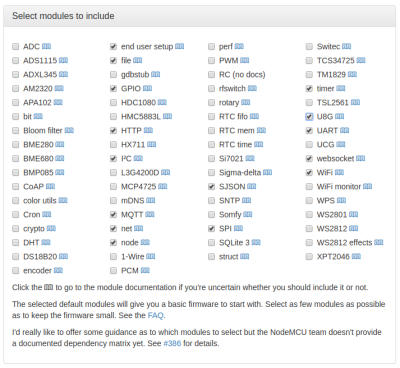
For my application, I needed to compile support in NodeMCU for a few more features than usual, so let’s over that in detail. The excellent NodeMCU-build tool will make this easy
Besides the default modules, I needed at least I2C, SPI, HTTP, websocket and SJSON. I also recommend adding MQTT and end user setup as well for a little flexibility.
There are a couple of optional graphics libraries to deal with LCD screens. Most of the screens available to me have fallen under the U8G module (or occasionally under the UCG module). When you select one of them, this tool provides options for fonts, as well as I2C and SPI display drivers. Fonts take up valuable space. My personal preference is to remove the two default choices (press the little ‘select’ link to open a menu) and choose u8g.font_profont17r and u8g.font_profont15r as both of those are easy to read and leave me two font size choices with a decent character set.
Finally, I selected display drivers from the dropdown listed as chipset/screensize/protocol and clicked the build button. I needed the float firmware and not the integer version — I don’t enough Ethereum to without decimal point values!
While waiting for it to build, I went to Etherscan and register for an API key. Once the firmware was built, I flashed it and we’re ready to go.
The Program
With hardware figured out, and modules added to the firmware, it’s time to write the code. I only needed four basic parts to make this happen: a network connection, some API calls, a way to show the data on the screen, and a timing mechanism to update at regular intervals.
WiFi:
We’ll start by connecting to Wi-Fi:
wifi.setmode(wifi.STATION) wifi.setphymode(wifi.PHYMODE_B) station_cfg={} station_cfg.ssid="my_ssid" station_cfg.pwd="my_password" station_cfg.save=true wifi.sta.config(station_cfg)
I’ve set PHYMODE_B to improve the range at the expense of power. I’m not using a battery, so figured I may as well have decent wireless range.
Retrieving Wallet Balance:
Next, we’ll define a function to retrieve the account balance of a specific wallet address. This will return the balance in Wei (1 Eth = 1,000,000,000,000,000,000 Wei). Replace ‘target address’ and ‘API key’ with your values.
function amount() http.get("http://api.etherscan.io/api?module=account&action=balance&address=target address&tag=latest&apikey=API key", nil, function(code, data) if (code < 0) then print("HTTP request failed") else print(code, data) t = sjson.decode(data) wei = (t["result"]) eth = wei/1000000000000000000 print (eth) end) end
Above, we see how neat the sjson library is. If you look at the raw JSON data the NodeMCU receives, it looks something like this:
Above, sjson.decode converts the received data into a table. Running t[“result”] returns the value associated with the field named ‘result’ (and we convert it from Wei to Eth). This is a very clean and easy way to deal with the many APIs that return JSON data!
Pushing Data to the OLED Display:
Next, we extend the function to display data on the screen.
function amount() http.get("http://api.etherscan.io/api?module=account&action=balance&address=target address&tag=latest&apikey=API key", nil, function(code, data) if (code < 0) then print("HTTP request failed") else print(code, data) t = sjson.decode(data) wei = (t["result"]) eth = wei/1000000000000000000 print (eth) -- Setup screen sda = 1 scl = 2 sla = 0x3C i2c.setup(0, sda, scl, i2c.SLOW) disp = u8g.ssd1306_128x32_i2c(sla) disp:setFontRefHeightExtendedText() disp:setDefaultForegroundColor() disp:setFontPosTop() disp:firstPage() repeat -- choose font disp:setFont(u8g.font_profont17r) -- display balance disp:drawStr(2, 22, eth) until disp:nextPage() == false end end) end
The Waiting Games:
Finally, we add a timer so Wi-Fi has time to connect before the function tries to retrieve your Eth balance, and then update every 10 seconds. There are more elegant ways to do this (e.g. run only once connected) but this works very well for the moment. Once I decide whether it will use a static Wi-Fi configuration or the end user configuration tool, I’ll likely change it to the latter method.
--wait 10 seconds for wifi to connect before trying to access the Eth balance tmr.alarm(0,10000,tmr.ALARM_AUTO,amount)
Build… Code… Profit!
There we have it! A simple device that retrieves and displays an Eth balance fresh from the blockchain. No, this is not my account, I used one from the API docs to see how it would actually handle large numbers.
There are many avenues this could be extended with new functionality, both frivolous and useful. Keep your eye on Hackaday as I’ll soon have a follow-up that implements something like that conference room rental concept. But I could totally make a screen that lets you pay Eth to rickroll me at work! What’s your best and worst idea for microcontrollers that interact with the blockchain?
You have 670456 Ethereum? You’re aware it’s worth USD$450 million, right?
From the article:
“No, this is not my account, I used one from the API docs to see how it would actually handle large numbers.”
Well, someone out there has 670456 Ethereum in his wallet, currently worth USD$448 million.
So this is what cryptocurrency has boiled down to: money. Who cares about the blockchain technology, as long as you can convince a bunch of newcomers to buy your line of SHA256s so that they can sell it to other newcomers/normies/hack-a-day-reader that is all that matters. Now if you excuse, I’m returning to /biz/, unironically.
I’ve been digging into private ethereum chains using state machines.
I’m having a hard time NOT believing that this will soon be 100% of the internet…
MetaMask let’s you use common browsers to interact. Private chains are free.
Darkweb will probably be first to move.
Example of, “if all you have is a hammer, every problem looks like a nail”.
You have a point here, and I’m often guilty of that approach too (NodeMCU for everything)… but I think it’s worth noting that you do sometimes find unexpected uses for hammers this way.
PS: state machines only place a hash of off-chain transactions on the blockchain, not transactions, so the space savings for the hard drive is exponential.
NO! Please don’t bring this fad into the wonderful world of microcontrollers.
Apropos:
https://www.youtube.com/watch?v=9nazm3_OXac
To be completely honest, I additionally don’t ask “Why would anyone even want to do that?”
Why fad? And why not?
Imagine using a private chain to connect every single traffic light in the city, and let each one have its own address. When you need to program one, you just send a transaction to its address, and the microcontroller will catch the change and reprogram itself. And you can know for sure who sent the change, and when. No more “it wasn’t me”, no more “we don’t know when this changed”, no need to physical access to update anything.
It’s not a fad, but it’s hyper-hyped for sure. Blockchain is not the cure for all and every disease, nuisance, issue and problem out there, but can be useful in a couple situations.
Because then microcontrollers will become scarce as they get scooped up for mining, just like GPUs!
Uhh, GPU’s get scooped up because they can mine certain protocols. Microcontrollers will never be fast enough, even in great numbers, to compete with juet one gpu. Also, GPU’s hit the second hand market quickly after many miners sell off their rigs when they find out its not as profitable as they hoped. Also, old mining gear gets replaced with newer stuff, so it goes to liquidation again. These are likely non overclocked cards, juet running at full load 100% of the time.
But can you imagine the blocksize for something like this?
Surely some transactions can be dropped after some time, it could even be part of the protocol itself, sort of like browser cookies.
Proof of Stake consensus and State Machines fix all of this.
Imagine waiting for the traffic to change every 3 minutes
State machines can have uint64 transactions per second, and only add a 32byte hash to the blockchain when it closes.
I’m pretty sure you could get some medication to help you deal with that kind of broken thinking process.
And what would be the value of a private chain again? Afaik the value of a blockchain is that it is a distributed consensus based system, so if you use a blockchain only for yourself you could just as well set up a normal database and save a lot of electricity because you don’t have to validate every transaction because you should already trust your own hard/software.
It is in effect a trusted computing platform. All changes are signed, and the fact that it operates on economic theory means that it costs more to hack than you make, so it’s secure.
Key pairs, encryption, non- fungible digital assets… if you put some time into reading about it, it becomes obvious that it is going to be the future of most of our internet. They can now do full SQL databases, c# interpreters, and so on. Business management and CRM software are obvious candidates as well.
https://medium.com/tag/ethereum
Business management chains with integrated TensorFlow for metrics analysis is the near future, I believe.
Can i have some of that stuff you guys are on?
I’m working to understand how to port spruned (https://github.com/gdassori/spruned) in micropython, to run in on the EPS8266. This would mean native blockchains on the ESP8266, without third party services.