We’ve devoted a fair amount of virtual ink here to casting shade at self-driving vehicles, especially lately with all the robo-taxi fiascos that seem to keep cropping up in cities serving as testbeds. It’s hard not to, especially when an entire fleet of taxis seems to spontaneously congregate at a single point, or all it takes to create gridlock is a couple of traffic cones. We know that these are essentially beta tests whose whole point is to find and fix points of failure before widespread deployment, and that any failure is likely to be very public and very costly. But there’s someone else in the self-driving vehicle business with way, WAY more to lose if something goes wrong but still seems to be nailing it every day. Of course, we’re talking about NASA and the Perseverance rover, which just completed a record drive across Jezero crater on autopilot. The 759-meter jaunt was completely planned by the onboard AutoNav system, which used the rover’s cameras and sensors to pick its way through a boulder-strewn field. Of course, the trip took six sols to complete, which probably would result in negative reviews for a robo-taxi on Earth, and then there’s the whole thing about NASA having a much bigger pot of money to draw from than any start-up could ever dream of. Still, it’d be nice to see some of the tech on Perseverance filtering down to Earth.
Hackaday Columns4114 Articles
This excellent content from the Hackaday writing crew highlights recurring topics and popular series like Linux-Fu, 3D-Printering, Hackaday Links, This Week in Security, Inputs of Interest, Profiles in Science, Retrotechtacular, Ask Hackaday, Teardowns, Reviews, and many more.
Horrendous Mess Of Wires
When do you post your projects? When they’re done? When they’re to the basic prototype stage? Or all along the way, from their very conception? All of these have their merits, and their champions.
In the post-all-along-the-way corner, we have Hackaday’s own [Arya Voronova], who outlines the many ways that you can start documenting your project before it’s even a fully fledged project. She calls these tidbits “breadcrumbs”, and it strikes me as being a lot like keeping a logbook, but doing it in public. The advantages? Instead of just you, everyone on the Internet can see what you’re up to. This means they can offer help, give you parts recommendations, and find that incorrect pinout that one pair of eyes would have missed. It takes a lot of courage to post your unfinished business for all to see, but ironically, that’s the stage of the project where you stand to gain the most from the exposure.
On the opposite end of the spectrum are the folks who document their projects at the very end. We see a ton of these on Hackaday.io and in people’s personal blogs. It’s a great service to the community, frankly, because at that point, you’re already done with the project. This is the point where the reward, for you, is at its minimum, but it’s also the point where you feel least inhibited about sharing if you’re one of those people who are afraid of showing your work off half-done. The risk here, if you’re like me, is that you’re already on to the next project when one is “done”, and going back over it to make notes seems superfluous. Those of you who do it regardless, we salute you!
And then there’s the middle ground. When you’re about one third of the way done, you realize that you might have something half workable, and you start taking a photo or two, or maybe even typing words into a computer. Your git logs start to contain more than just “fixed more stuff” for each check-in, because what if someone else actually reads this? Maybe you’re to the point where you’ve just made the nice box to put it in, and you’re not sure if you’ll ever go back and untangle that rat’s nest, so you take a couple of pictures of the innards before you hot glue it down.
I’m a little ashamed I’m probably on the “post only when it’s done” end of things than is healthy, mostly because I don’t have the aforementioned strength of will to go back. What about you?
Retrotechtacular: How Communism Made Televisions
For those of us who lived through the Cold War, there’s still an air of mystery as to what it was like on the Communist side. As Uncle Sam’s F-111s cruised slowly in to land above our heads in our sleepy Oxfordshire village it was at the same time very real and immediate, yet also distant. Other than being told how fortunate we were to be capitalists while those on the communist side lived lives of mindless drudgery under their authoritarian boot heel, we knew nothing of the people on the other side of the Wall, and God knows what they were told about us. It’s thus interesting on more than one level to find a promotional film from the mid 1970s showcasing VEB Fernsehgerätewerk Stassfurt (German, Anglophones will need to enable subtitle translation), the factory which produced televisions for East Germans. It provides a pretty comprehensive look at how a 1970s TV set was made, gives us a gateway into the East German consumer electronics business as a whole, and a chance to see how the East Germany preferred to see itself.
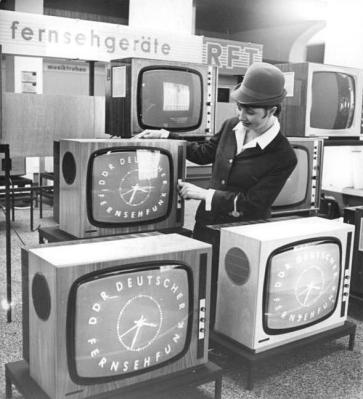
The sets in question are not too dissimilar to those you would have found from comparable west European manufacturers in the same period, though maybe a few things such as the use of a tube output stage and the lack of integrated circuits hints at their being a few years behind the latest from the likes of Philips or ITT by 1975. The circuit boards are assembled onto a metal chassis which would have probably been “live” as the set would have derived its power supply by rectifying the mains directly, and we follow the production chain as they are thoroughly checked, aligned, and tested. This plant produces both colour and back-and-white receivers, and since most of what we see appears to be from the black-and-white production we’re guessing that here’s the main difference between East and West’s TV consumers in the mid ’70s.
The film is at pains to talk about the factory as a part of the idealised community of a socialist state, and we’re given a tour of the workers’ facilities to a backdrop of some choice pieces of music. References to the collective and some of the Communist apparatus abound, and finally we’re shown the factory’s Order of Karl Marx. As far as it goes then we Westerners finally get to see the lives of each genosse, but only through an authorised lens. Continue reading “Retrotechtacular: How Communism Made Televisions”
Hackaday Podcast 238: Vibrating Bowl Feeders, Open Sourcery, Learning To Love Layer Lines
Elliot Williams and Tom Nardi start this week’s episode off with some deep space news, as NASA’s OSIRIS-REx returns home with a sample it snapped up from asteroid Bennu back in 2020. From there, discussion moves on to magical part sorting, open source (eventually…) plastic recycling, and the preposterously complex method newer Apple laptops use to determine if their lid is closed. They’ll also talk about the changing perceptions of 3D printed parts, a new battery tech that probably won’t change the world, and a clock that can make it seem like your nights are getting longer and longer. Stick around until the end to hear about the glory days of children’s architecture books, and the origins of the humble microwave oven.
Check out the links below if you want to follow along, and as always, tell us what you think about this episode in the comments!
This Week In Security: Magic Packets, GPU.zip, And Enter The Sandman
Leading out the news this week is a report of “BlackTech”, an Advanced Persistent Threat (APT) group that appears to be based out of China, that has been installing malicious firmware on routers around the world. This firmware has been found primarily on Cisco devices, and Cisco has released a statement clarifying their complete innocence and lack of liability in the matter.
It seems that this attack only works on older Cisco routers, and the pattern is to log in with stolen or guessed credentials, revert the firmware to a yet older version, and then replace it with a malicious boot image. But the real fun here is the “magic packets”, a TCP or UDP packet filled with random data that triggers an action, like enabling that SSH backdoor service. That idea sounds remarkable similar to Fwknop, a project I worked on many years ago. It would be sort of surreal to find some of my code show up in an APT.
Don’t Look Now, But Is Your GPU Leaking Pixels
There’s a bit debate on who’s fault this one is, as well as how practical of an attack it is, but the idea is certainly interesting. Compression has some interesting system side effects, and it’s possible for a program with access to some system analytics to work out the state of that compression. The first quirk being leveraged here is that GPU accelerated applications like a web browser use compression to stream the screen view from the CPU to the GPU. But normally, that’s way too many pixels and colors to try to sort out just by watching the CPU and ram power usage.
And that brings us to the second quirk, that in Chrome, one web page can load a second in an iframe, and then render CSS filters on top of the iframe. This filter ability is then used to convert the page to black and white tiles, and then transform the white tiles into a hard-to-compress pattern, while leaving the black ones alone. With that in place, it’s possible for the outer web page to slowly recreate the graphical view of the iframe, leaking information that is displayed on the page.
And this explains why this isn’t the most practical of attacks, as it not only requires opening a malicious page to host the attack, it also makes some very obvious graphical changes to the screen. Not to mention taking at least 30 minutes of data leaking to recreate a username displayed on the Wikipedia page. What it lacks in practicality, this approach makes up for in cleverness and creativity, though. The attack goes by the GPU.zip moniker, and the full PDF is available. Continue reading “This Week In Security: Magic Packets, GPU.zip, And Enter The Sandman”
The Oldest Living Torrent Is 20 Years Old
Twenty years ago, in a world dominated by dial-up connections and a fledgling World Wide Web, a group of New Zealand friends embarked on a journey. Their mission? To bring to life a Matrix fan film shot on a shoestring budget. The result was The Fanimatrix, a 16-minute amateur film just popular enough to have its own Wikipedia page.
As reported by TorrentFreak, the humble film would unknowingly become a crucial part of torrent history. It now stands as the world’s oldest active torrent, with an uptime now spanning a full 20 years. It has become a symbol of how peer-to-peer technology democratized distribution in a fast-changing world.
Continue reading “The Oldest Living Torrent Is 20 Years Old”
You’ve Got Mail: Faster And Faster We Go
When we last left the post office, they had implemented OCR to read even the sloppiest of handwriting. And to augment today’s 99% accuracy rate, there’s a center full of humans who can decipher the rest of those messy addresses with speed and aplomb. Before that, we took a look at many of the machines that make up the automated side of the post office’s movements. But what was being done to improve the customer experience during all of this time?
Quite a bit, as it turns out. In this installment, we’ll take a look at the development of vending machines and programs like Speed Mail, Missile Mail, and V-Mail (no, not voicemail!) as they relate to enhanced customer service over the years.